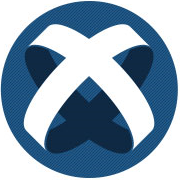
Like Xamarin, Titanium is a hybrid solution that facilitates use of a familiar language and generates native apps. We made the point in Part 6 that any hybrid solution you're considering should be carefully examined to assess cost, performance, reliability, and risk.
Titanium: Mobile Development in JavaScript
Appcelerator Titanium is a leading hybrid mobile platform. JavaScript is arguably the most popular programming language on planet earth today--anyone who does web development has had to learn it, and the language hasn't stopped there: thanks to node.js, it is also being used server-side. It makes sense, therefore, to make mobile app development accessible to the world's large numbers of JavaScript developers. That's what Titanium does. To quote the Titanium web site, Titanium is "An open, extensible development environment for creating beautiful native apps across different mobile devices and OSs including iOS, Android, Windows and BlackBerry, as well as hybrid and HTML5. It includes an open source SDK with over 5,000 device and mobile operating system APIs, Studio, a power Eclipse-based IDE, Alloy, an MVC framework and Cloud Services for a ready-to-use mobile backend."
Titanium generates native apps that execute a JavaScript interpreter at runtime, but a very efficient one, paired with native objects in the mobile platform's runtime environment (see What Titanium Appcelerator Really is and How it Works). This is different from executing within a browser, the approach taken by PhoneGap and Icenium.
Titanium development can be fee or paid, depending on how much of Appcelerator's services you are interested in taking advantage of. From the web site: "The free version of Titanium is primarily a solution for the development of mobile apps, whereas the Appcelerator Platform supports the entire mobile lifecycle, along with commercial support, SLAs and technical training. The Appclerator Platform includes Appcelerator Studio, Appcelerator Alloy, Appcelerator Cloud, Appcelerator Test, Appcelerator Analytics, and Appcelerator Performance Management." (please review Plans and pricing and this forum thread).
The Computer You'll Develop On: Windows, Mac, or Linux
Titanium's IDE, Titanium Studio, is available in Windows, Mac, and Linux editions, giving you a lot of choice in your development machine. You'll be needing a Mac if you intend to do iOS development.
Developer Tools: Titanium Studio
The Titanium Studio IDE is based on Eclipse. A single solution can contain projects for multiple mobile targets, which according to Appcelerator leads to 60-90% code reuse.
Depending on type of computer and whether you have previously installed the SDKs for your target mobile platforms, installing and configuring Titanium may be a breeze or it may have you pulling out your hair--it can take a bit of work before you've got everything in place and the right paths and environment variables set up. The Mac setup appears to be the most streamlined in my experience. There's a Getting Started dashboard in Titanium Studio that works to help with all this.
Titanium Studio - Dashboard
Titanium Studio - on a Mac
Debugging Experience
As you would expect, at runtime you can run in a mobile platform emulator, or an actual device connected to your computer.
For iOS devices, getting your app deployed to an iPhone or iPad requires registering your app with the Apple developer center (a standard requirement) and then deploying through iTunes or XCode and syncing with your device--these are some extra steps you don't have to deal with in other mobile development environments, which some may find cumbersome.
For Android devices, things are a bit simpler: you can deploy and run right out of Titanium Studio.
Programming: JavaScript
Here's a fragment of Titanium JavaScript code (from Seven Days with Titanium).
var table1 = Titanium.UI.createTableView({
style:Titanium.UI.iPhone.TableViewStyle.GROUPED
});
var section1 = Titanium.UI.createTableViewSection();
section1.headerTitle = "Hello";
var row1 = Titanium.UI.createTableViewRow({title:"Hello 1"});
var row2 = Titanium.UI.createTableViewRow({title:"Hello 2"});
section1.add(row1);
section1.add(row2);
var section2 = Titanium.UI.createTableViewSection();
section2.headerTitle = "Hello2";
var row3 = Titanium.UI.createTableViewRow({title:"Hello 3"});
var row4 = Titanium.UI.createTableViewRow({title:"Hello 4"});
section2.add(row3);
section2.add(row4);
table1.setData([section1,section2]);
Here's how this code renders on iOS and Android:
Here's another code example from the same blog series that shows playing video:
var videoURL = 'http://movies.apple.com/media/us/ipad/2010/tours/apple-ipad-video-us-20100127_r848-9cie.mov';
var activeMovie = Titanium.Media.createVideoPlayer({
url: videoURL,
width:300,
height:200,
top:50,
left:50,
backgroundColor:'#0f0'
});
view.add(activeMovie);
activeMovie.play();
Capabilities
Titanium allows you good access to mobile platform APIs. Your code can interact with device sensors like the camera, GPS, and accelerometer; and get to resources like Photos, Contacts, and Maps.
Titanium KitchenSink Sample showing Map Access on iOS
Online Resources
The Appcelerator Developer Center includes downloads. documentation, training, and community forums.
Key resources:
Quick Start Guide
Training Resources
Sample Code
Appcelerator Cloud Services
Appcelerator offers Appcelerator Cloud Services, which provide Mobile Backend as a Service (MBaaS) functionality. Services provided include push notification, status updates, photo storage, and social integration.
TutorialsAppcelerator provides both free training resources as well as paid training classes. Titanium also has a certified developer program.
Creating Your First Titanium Apps
This initial tutorial gets you oriented with Titanium and shows how to import sample projects; and also how to create a custom project.
KitchenSink Sample running on Android and iOS
Tutorial App running on Android and iOS
Building Native Mobile App with Titanium
This series of tutorials follows the Titanium Certified App Developer (TCAD) course, an introductory training course. They are fairly sparse, not providing a lot of detail.
1a. Native Android Development
1b. Native iPhone Development
These labs guide you through creating, building, and running a simple "Hello, World" app using native Android and iOS tools.
2. Cross-Platform Mobile Development in Titanium
This lab has you begin creation of a cross-platform mobile app called TiBountyHunter, which enables bounty hunters to track a list of fugitives on their mobile device.
Lab 2 - TiBountyHunter
3. UI Fundamentals
This lab has you create the user interface for TiBountyHunter, including table views and windows. populated with dummy data.
Lab 3 - TiBountyHunter with UI and Dummy Data
4. Working with Local Data
This lab uses data from a local SQL database to populate the app's fugitive list.
Lab 4 - TiBountyHunter with data from local SQL Database
5. Working with Remote Data
This lab enhances the TiBountyHunter app to retrieve a list of fugitives from a remote web service.
Lab 5 - TiBountyHunter - with data retrieved from a Web Service
6. Working with Media and the File System
In this lab, you use the camera to add an image to your fugitive and save the image to the file system.
Lab 6 - Camera Support
7. Using Location ServicesIn this lab, you add geolocation support to TiBountyHunter, storing the coordinates of where a fugitive is captured and providing an option to plot location on a map.
Lab 7 - Location Services
8. Appcelerator Cloud Services
In this lab, you integrate Appcelerator Cloud Services so users can boast about their captured bounties.
Lab 8 - Integrated with Cloud Services
9. Integrating Web Content
In this lab, you integrate web content into a partial RSS reader.
Lab 9 - RSS reader
10. Deploy and Distribute
In this lab, you deploy an app for testing on a physical device and follow steps for publishing to the Android Market and iTunes App Store.
Contrasting Xamarin and Titanium
It's hard not to draw parallels between Titanium and Xamarin because they have many similarities. Like Xamarin, Titanium is all about using a familiar language--JavaScript in this case--to do your mobile development. Like Xamarin, Titanium generates native applications and gives your code access to the APIs of your target mobile platforms. Both host an execution layer in their native apps (the Google V8 JavaScript engine for Titanium, Mono/.NET for Xamarin). Titanium has been around a few years longer than Xamarin.
Although they fill the same need and have similarities, it's important to evaluate them individually. If you've decided on a hybrid approach and are torn between the two, take the time to get familiar with each of them and you'll likely find reasons to favor one over the other.
Summary: Titanium Hybrid Mobile Development in JavaScript
Titanium puts mobile development in easy reach of JavaScript developers, the world's largest programmer community. While it's still necessary to learn the APIs and conventions of each mobile platform, taking Objective C and Java out of the equation is nevertheless a major boost. And, being able to use a common language for your iOS and Android projects makes a good deal of code re-use possible.
Next: Getting Started with Mobility, Part 8: Hybrid Development in HTML5 with Icenium
No comments:
Post a Comment